· web development · 6 min read
What are the Differences between Functional and Class-Components in React?
This article's goals are to explain the differences between functional and class components in React and to offer advice on when to use each.
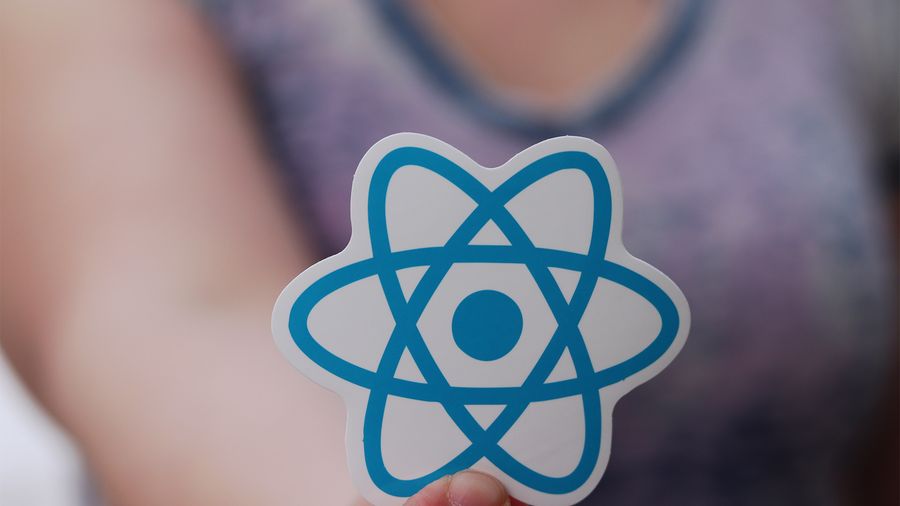
The fundamentals of building and using React components are covered on this page, along with information on using props to pass data across components. The documentation also covers more complex subjects like using hooks in functional components and state and lifecycle methods in class components. The documentation also offers a ton of examples and code snippets to assist users in learning how to use React components and properties.
By encapsulating the structure and functionality of UI elements, React components allow for modularity and reusability in online applications. The simplest and lightest approach to define a component in React is with functional components. They are ideal for straightforward UI elements without any state constraints.
React hooks increase the flexibility and power of functional components, whereas class components offer more advanced capabilities, such as state management. Overall, functional components are a fantastic place to start when building React apps, but additional component kinds and technologies are also available for building more sophisticated and potent programs.
function Greetings(props) {
return <h1>Welcome, {props.name}</h1>;
}
React components can be defined in two ways: using a function with props as input (functional components) or using the ES6 class syntax (class components). Functional components are quick and easy to create and are best for simple presentational components, while class components can handle state and have more complex behavior.
They can also be used to build customizable component libraries. Overall, React provides a flexible and powerful way to generate reusable building blocks for your user interface.
class Greetings extends React.Component {
render() {
return <h1>Welcome, {this.props.name}</h1>;
}
}
When deciding whether to use functional or class components in React, it’s important to consider the demands of your project. Functional components are simple and fast, making them a good choice for straightforward components without state management or lifecycle methods. Class components are more complex but can handle state and behavior in a single component, making them a good choice for more complicated components. Ultimately, the decision depends on the specific requirements of your component and your project goals. It’s imkillconportant to understand the advantages and disadvantages of each approach and choose the one that best fits your needs.
Functional vs. Class Components in React and Syntax and Code Differences
One of the main differences between functional and class components in React is their syntax. Functional components are written as plain JavaScript functions that take in props as an argument and return a React element. On the other hand, class components require you to extend the React.Component class and define a render function that returns a React element. While this may require more code, it also provides additional benefits that will be discussed later.
Furthermore, if you examine the transpiled code using Babel, you’ll notice significant differences between the two types of components.
State in React
React’s functional elements are essentially just JavaScript functions with a return value of a React element. They were unable to control their own state using the “setState” method in early iterations of React. But, functional components can now manage state thanks to the “useState” hook.
Use the “useState” hook to establish state variables if your functional component needs to manage state. This eliminates the requirement to change your functional component into a class component and allows you to manage and update state within it.
import React, { useState } from 'react';
function Greetings({ name }) {
const [count, setCount] = useState(0);
const incrementCount = () => setCount((prevCount) => prevCount + 1);
return (
<div>
<h1>Welcome, {name}</h1>
<p>
You have visited us <span>{count}</span> times.
</p>
<button onClick={incrementCount}>Increment</button>
</div>
);
}
With the “useState” hook, we defined the state variable “count” in this example. The “setCount” function can then be used to update the count variable. By doing this, we can manage state within our functional component and maintain clear, understandable code.
Lifecycle Hooks vs. Lifecylce Events
Lifecycle events and hooks in React describe the various stages of a component’s existence from the time it is generated until it is deleted from the DOM. You may regulate and alter the behavior of your components at various points in their lifespan thanks to these hooks and events.
A React component’s lifecycle comprises three key stages: mounting, updating, and unmounting. There are events and hooks connected to each of these phases that you may use to carry out various tasks.
When a component is first generated and added to the DOM, it enters the mounting phase. The following hooks and events are accessible during this stage:
- The first hook to be called during the Mounting phase is
constructor()
. It is used to bind methods to the component’s instance and set the component’s initial state. - Next, the
render()
hook is used to create the JSX that will be added to the DOM. componentDidMount()
: This hook is invoked upon the addition of the component to the DOM. Any essential setup, such as obtaining data or configuring event listeners, is carried out using it.
When the state or props of a component are modified, the updating phase starts. The following hooks and events are accessible during this stage:
- Should the component be re-rendered? May be decided by calling the hook
shouldComponentUpdate()
before the component is updated. - To create the modified JSX that will be appended to the DOM, the
render()
hook is called. componentDidUpdate()
: This hook is invoked upon an update to the component. It is used to carry out any required cleanup or further setup, such changing the component’s state or obtaining fresh data.
When a component is taken out of the DOM, it enters the unmounting phase. The following hooks and events are accessible during this stage:
componentWillUnmount()
: Just before the component is removed from the DOM, this hook is invoked. It is utilized to carry out any necessary cleanup, including the elimination of event listeners and the termination of any pending network requests.
You can better control the behavior of your components and make sure they are effective and performant by being aware of the many lifecycle hooks and events React offers. You may build dynamic, responsive web applications that offer a smooth user experience by wisely utilizing these hooks and events.
Therefore why would I even consider using functional components?
You might wonder why, since functional components take away so many desirable features, you should use them at all. However utilizing functional components in React has certain advantages as well:
- Functional components are straightforward JavaScript functions without state or lifecycle-hooks, making them more simpler to comprehend and test.
- They assist you in employing optimal practices. If your component doesn’t have access to setState(), it will become simpler to distinguish between presentational and container components since you will need to consider your component’s state more.
- Future versions of React may offer a speed boost for functional components, according to the React team.
Conclusion
React’s functional and class components each offer advantages and disadvantages. Class components can handle state management and lifecycle operations, but functional components are simpler to read and test, produce less code, and promote best practices. Consider the particular demands of your project as well as the specifications of your components when choosing the sort of component to utilize. In the end, your project’s unique requirements and the functionality you need will determine whether you choose functional or class components.