· engineering · 14 min read
How to Start Using Git and GitHub? - A Step-by-Step Guide for Newcomers
Are you new to Git? To get comfortable with making modifications to the code base, opening pull requests (PRs), and merging code into the primary branch.
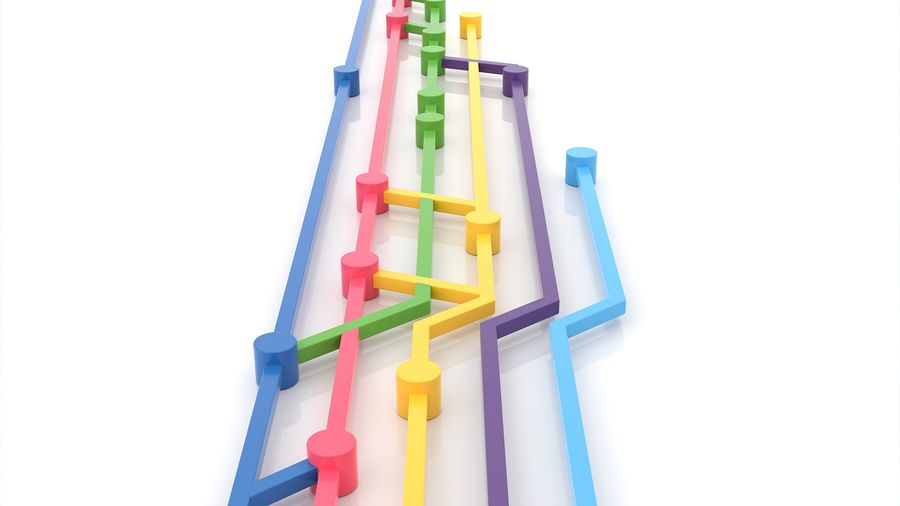
Get Git set up on your computer and establish a GitHub account to get started
Before you get started, there are two essential steps to take: installing Git and setting up a GitHub account.
To install Git, follow the instructions found here. For the purpose of this tutorial, we will be working solely with the Git command line interface. Although there are several Git graphical user interfaces available, it’s recommended to start by learning Git with its specific commands before exploring a GUI. Keep in mind that the vast majority of online Git resources and discussions will also be for the command line.
Once you’ve completed the Git installation, create a GitHub account using this link.
Git and GitHub
Before we move forward, it’s important to understand the difference between Git and GitHub. Git is an open-source version control tool developed by Linux developers in 2005, while GitHub is a company founded in 2008 that provides Git-integrated tools. Although GitHub is not a requirement for using Git, it is necessary to use Git in order to utilize GitHub. There are other alternatives to GitHub such as GitLab, BitBucket, and self-hosted solutions like gogs and gittea, all of which are referred to as “remotes” in Git terminology and are optional. While using a remote can make sharing your code easier, it is not required to use Git.
Establish a local Git repository on your machine
When starting a new project on your local machine with Git, the first step is to create a repository.
To use Git, we’ll be working in the terminal. If you’re new to the terminal and basic commands, take a look at this tutorial.
To get started, open your terminal and navigate to the desired location for your project using the cd (change directory) command. For example, if you have a projects folder on your desktop, you would do something like this:
$ cd ~
$ mkdir project
$ cd project
To set up a Git repository in the root directory of your folder, run the following command:
$ git init
Initialized empty Git repository in /home/user/project/.git/
Adding new file to the repository
Create a new file in the project using any text editor of your choice or by running a touch command, such as touch README.md to create an empty file named README.md.
After adding or modifying files in a folder with a Git repository, Git will recognize the new file. However, Git will not keep track of the file until you explicitly instruct it to do so. Git only records changes to files that it tracks, so you need to send a command to confirm that you want Git to keep track of your new file.
$ touch README.md
Once you’ve created the new file, you can use the git status command to view which files are known to Git.
$ git status
On branch master
No commits yet
Untracked files:
(use "git add <file>..." to include in what will be committed)
README.md
nothing added to commit but untracked files present (use "git add" to track)
In essence, this means that Git has detected the creation of a new file called README.md, but unless you use the git add command, Git will not take any action with it.
A brief intermission: Understanding the staging environment, committing, and you
One of the most challenging aspects of learning Git is grasping the concept of the staging environment and its relationship with committing.
A commit is a record of all the changes you’ve made since your last commit. Essentially, you make changes to your repository (such as adding or modifying a file), and then tell Git to put those changes into a commit.
Commits are the backbone of your project and allow you to revisit the state of the project at any given commit.
So, how do you tell Git which files to include in a commit? This is where the staging environment or index comes in. As mentioned, when you make changes to your repository, Git recognizes the changes, but won’t do anything with them until you add them to the staging environment.
To add a file to a commit, you first need to place it in the staging environment using the git add filename command.
Once you’ve used the git add command to place all the desired files in the staging environment, you can then tell Git to package them into a commit using the git commit command.
Add a file in the staging environment
Use the git add command to add a file to the staging environment.
After running the git add command, rerun the git status command to see that Git has added the file to the staging environment.
$ git add README.md
$ git status
On branch master
No commits yet
Changes to be committed:
(use "git rm --cached <file>..." to unstage)
new file: README.md
To emphasize, the file has not yet been recorded in a commit, but it’s now in the process of being committed.
Make a commit
It’s now time to finalize your first commit.
Execute the following command: git commit -m “Your commit message description”.
$ git commit -m "Your commit message description"
[master (root-commit) 882db5a] Your commit message description
1 file changed, 0 insertions(+), 0 deletions(-)
create mode 100644 README.md
The message accompanying the commit should provide context on the changes being made, whether it’s a new feature, bug fix, or even a simple typo correction. Avoid using nonsensical messages like “foo” or “bar” as they can be confusing to anyone reviewing your commit in the future. Commits remain in the repository permanently (although they can be removed in exceptional cases), so including a clear explanation of your changes can help future programmers, including yourself, understand the purpose of a change long after it was made.
Your first branch
Having made a commit, let’s delve into a more advanced aspect of Git branches.
Branches provide a convenient way to switch between different stages of a project. As per the official Git documentation, a branch in Git is essentially a lightweight, movable reference to one of these commits. For instance, if you want to add a new page to your website, you can create a separate branch for that page without affecting the main project. Once you’ve completed the page, you can merge your changes from that branch into the main branch. When you create a new branch, Git keeps track of the commit it was created from, allowing it to keep track of the history of all the files.
Suppose you’re on the main branch and you want to create a new branch to develop your web page. This is how you proceed:
$ git checkout -b awesome-branch
Switched to a new branch 'awesome-branch'
This command will automatically create a new branch and then ”check you out” on it, meaning Git will move you to that branch, away from the main branch.
After running this command, you can use the git branch command to confirm that your branch was created:
* awesome-branch
master
The branch with the asterisk * next to its name indicates the current branch you are on.
A side note on naming conventions for branches
It’s worth mentioning that the default name for the first branch in a Git repository is typically master. This branch is often used as the primary branch in a project. However, some groups have started using alternative names for the default branch in response to anti-racism efforts in the tech industry. For example, in this tutorial, we use the term “primary” instead of “master”. In other documentation and discussions, you may come across the use of “master” or other terms to refer to the main branch. Regardless of the name, it’s important to keep in mind that most repositories have a primary branch that represents the official version of the repository. For websites, this is the version that users see. For applications, this is the version that users download. While this distinction is not technically necessary in Git, it is a common convention in project management.
For those interested in the reasoning behind the use of alternative default branch names, GitHub provides an explanation of their change at this link: https://github.com/github/renaming.
If you were to return to the primary branch and make further commits, your new branch would remain unaffected until you incorporate those changes through merging.
Setting up a new repository on GitHub
Collaborating with a team through GitHub.
While you can use Git to keep track of your code locally, using GitHub allows you to work with others on the same project. To set up a new repository on GitHub, simply log in to your account and navigate to the GitHub homepage. You’ll find the “New repository” option located under the ”+” sign next to your profile picture in the top right corner of the navigation bar.
Upon clicking the button, GitHub will prompt you to give your repository a name and provide a brief description.
After completing the information, hit the “Create repository” button to establish your new repository.
GitHub will then ask if you want to create a new repository from scratch or if you have an existing local repository you’d like to add. As we have already created a local repository, we’ll follow the instructions under the “…or push an existing repository from the command line” section.
$ git remote add origin https://github.com/IshtarStar/repository.git
$ git push -u origin master
Counting objects: 1, done.
Writing objects: 100% (1/1), 418 bytes | 0 bytes/s, done.
Total 1 (delta 0), reused 0 (delta 0)
To https://github.com/IshtarStar/repository.git
* [new branch] master -> master
Branch master set up to track remote branch master from origin.
Sending a branch to GitHub using “push”
The next step is to share the changes in your branch with the rest of the team by pushing it to your new GitHub repository. This makes it possible for others to view your changes and, if approved by the repository owner, merge them into the primary branch.
Share your changes with the team by pushing them to your new GitHub repository by running the command git push origin awesome-branch. This will automatically establish the branch on the remote repository on GitHub.
$ git push origin awesome-branch
Counting objects: 1, done.
Delta compression using up to 4 threads.
Compressing objects: 100% (1/1), done.
Writing objects: 100% (1/1), 418 bytes | 0 bytes/s, done.
Total 1 (delta 0), reused 0 (delta 0)
To https://github.com/IshtarStar/repository.git
* [new branch] awesome-branch -> awesome-branch
The word “origin” in the command git push origin awesome-branch is an alias created by git when you clone a remote repository to your local machine. This alias refers to the URL of the remote repository and is usually named ”origin.” Instead of using the full URL to push changes, you can use this alias. For example, you can use either git push git@github . com:IshtarStar/repository.git awesome-branch or git push origin awesome-branch.
If you visit the GitHub repository page, you’ll notice that a notification indicating that a new branch with your specified name has been pushed. You can also access the list of branches by clicking the “branches” link on the page.
Hit the button Compare & pull request.
Create your first PR (Pull Request)
A pull request (PR) is a mechanism used to notify the owners of a code repository that you intend to make modifications to their code. It enables them to examine and verify the code to ensure that it is in good shape before merging your changes into the primary branch.
At times, you may come across a conspicuous green button at the bottom of a pull request, labeled ‘Merge pull request’. By clicking this button, you can integrate the changes you have proposed into the primary branch.
If you are the sole owner or a co-owner of a repository, creating a pull request may not always be necessary to merge your changes. However, it is still recommended to make one, as it enables you to maintain a comprehensive history of your updates and also ensures that you always work on a new branch when making changes.
Merge your pull request (PR)
Feel free to proceed and click the green button labeled ‘Merge pull request’. This action will integrate the changes you’ve made into the primary branch.
After you’ve merged the pull request, I highly recommend that you delete your branch. This is because having too many branches can become messy over time. To delete your branch, click the gray button labeled ‘Delete branch’.
To double-check whether your commits have been merged, navigate to the ‘Commits’ link on the first page of your new repository. Here, you’ll find a list of all the commits in that branch. The one you just merged should be right at the top, with the label ‘Merge pull request #1’.
You’ll also see a hash object on the right-hand side of the commit. A hash object is a unique identifier for that specific commit. It can come in handy when referring to specific commits or when undoing changes. You can use the command git revert to backtrack.
Retrieve changes from GitHub to your local computer
At present, the repository on GitHub may look somewhat different than what you have on your local machine. For instance, the commit you created in your branch and merged into the primary branch might not exist on the primary branch of your local machine.
To obtain the most recent changes that you or others have merged on GitHub, utilize the command “git pull origin master” (when operating on the primary branch). This command can usually be abbreviated to just “git pull”.
$ git pull origin master
remote: Counting objects: 1, done.
remote: Total 1 (delta 0), reused 0 (delta 0), pack-reused 0
Unpacking objects: 100% (1/1), done.
From https://github.com/IshtarStar/repository.git
* branch master -> FETCH_HEAD
c937a6b..6758c5b master -> origin/master
Merge made by the 'recursive' strategy.
README.md | 1 +
1 file changed, 1 insertion(+)
This command will display a list of all the modified files and the changes that have been made to them.
After reviewing the changes, you can use the git log command once again to view the list of new commits.
$ git log
commit 92a1da597f09e8e02a2ac63093983d3f7c6c468c
Merge: 2c5ac71 3542c6a
Author: Marc Wolf <github@marc.it>
Date: Tue Feb 14 21:15:10 2023 +0100
Merge branch 'master' of https://github.com/IshtarStar/repository.git
commit dea2162da789d3d56eab0952d4f7a23479dd0cc4
Author: Marc Wolf <github@marc.it>
Date: Tue Feb 14 18:33:41 2023 +0100
Brand new file added
commit a2c9690c2935353ad69fc6a35c9fcfd4991e1bec
Merge: b345d9a 1e8dc08
Author: Marc Wolf <github@marc.it>
Date: Mon Feb 13 16:13:22 2023 +0100
Merge pull request #2 from IshtarStar/awesome-branch
Much more markdown descriptions
commit 8ce306cfffdc9cbbe6123ca8f2bb8a989a36ef84
Author: Marc Wolf <github@marc.it>
Date: Mon Feb 13 12:02:47 2023 +0100
More text into README.d
commit 74387648e341c4c16998dbe8e7dd6dd5b224dccf
Author: Marc Wolf <github@marc.it>
Date: Mon Feb 13 10:11:06 2023 +0100
Your commit message description
Take a moment to appreciate your success with Git
Congratulations on successfully creating a pull request and integrating your code into the primary branch! If you’re interested in learning more about Git, you may find the following advanced tutorials and resources to be useful:
- https://training.github.com/ GitHub’s official Git reference guides! They’re helpful for recalling the common commands you’ll use frequently.
- https://www.w3schools.com/git/ Git and GitHub are different things. In this tutorial you will understand what Git is and how to use it on the remote repository platforms, like GitHub.
- https://git-scm.com/docs/gittutorial This tutorial explains how to import a new project into Git, make changes to it, and share changes with other developers.
I also suggest that you set aside some time to collaborate with your team and undertake a mock group project like the one we just did. Create a new folder with your team name, and add some text files to it. Next, try pushing those changes to the remote repository. This way, your team can practice making changes to files they didn’t initially create and become familiar with utilizing the PR feature. Additionally, you can use GitHub’s git blame and git history tools to monitor changes made to a file and identify who made those changes.
The more you use Git, the more comfortable you’ll become with it. So, don’t hesitate to experiment and learn through hands-on experience.