· web development · 13 min read
What are the differences between Next.js and React and how can you decide which framework to choose for your project?
In this post, we'll delve into two of the most frequently employed JavaScript frameworks, React and Next.js, outlining the pros and cons of each to help you make an informed decision.
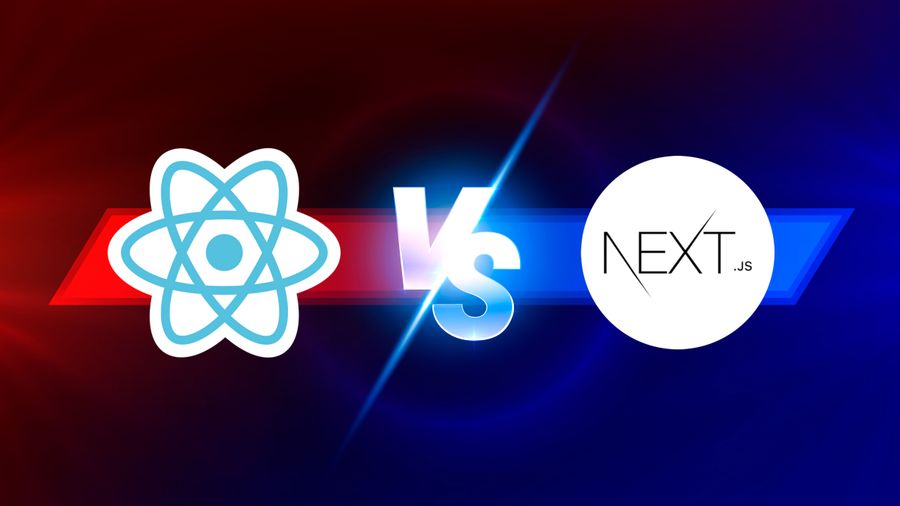
The popularity of React has made it the preferred framework for new developers in the JavaScript ecosystem. Next.js, which is built with the same React library as React, remains a viable alternative. This article explores the differences between the two frameworks and weighs the advantages and disadvantages one has over the other. In the end, you will have enough information to make an informed decision on which framework suits your project’s needs.
What Is React?
Meta developed React as a user interface library for building reactive applications that rely on event triggers. In traditional programming, when data needs to change, a website has to reload, leading to slower page rendering times whenever a user clicks on something.
However, with React’s use of components, the code or logic for a specific page is no longer being reprocessed. Components can be stateful or stateless, and they only re-render within the applied state’s scope.
React has a declarative architecture, granting users control over their workflow. By putting the user in charge of how the app operates, React becomes an incredibly powerful tool.
Essential Features of React
These features give React a leg up over competing frameworks:
- JSX JavaScript Syntax Extension
- One-way data binding
- Component
- Virtual DOM
JavaScript Syntax Extension
JavaScript Syntax Extension” is a feature in programming languages that allows developers to write code using a syntax similar to JavaScript. The use of syntax extensions enhances code functionality and makes it easier to read and write. TypeScript adds type annotations that help catch errors during compilation, while JSX allows HTML-like syntax within JavaScript, useful for creating user interface components with React. CoffeeScript compiles to JavaScript while reducing code redundancy. These languages all make use of JavaScript syntax and aim to improve code quality, readability, and maintainability.
import React, { useState } from 'react';
const ItemList = () => {
const [items, setItems] = useState(['Item 1', 'Item 2', 'Item 3']);
return (
<div>
<h2>My Items:</h2>
<ul>
{items.map((item) => (
<li key={item}>{item}</li>
))}
</ul>
</div>
);
};
export default ItemList;
One-Way Data Binding
In React.js, a component’s logic is responsible for determining the data that is displayed on the user interface. The connection between this data and the component is known as data binding.
React.js implements one-way binding as a simpler way to handle data flow between parent and child components. This method ensures that data flows from parents to children, but not the other way around, which can be a more complicated process.
In this one-way flow of data, the parent component passes information to the child component using what is called a “read-only prop.” This prop allows data to be passed down to child components, but it cannot be used to send data back to the parent. However, if the child component receives user input or other interactions that require state changes, it can still communicate with the parent component, allowing for a smooth and streamlined experience for users.
Component
React operates by breaking down an application’s user interface into smaller components. With React, every aspect of a user interface is treated as a component, even for the largest and most complicated applications. These individual pieces of a user interface can be combined to create intuitive and straightforward user experiences.
Every component in React can have its logic and behaviors, making it a powerful and versatile tool in the development process. With reusable components, developers can quickly create similar user interfaces without reinventing the wheel for every piece of an application.
This versatility allows React components to be reused in any part of a web page, just by calling them. This powerful feature makes React virtually limitless in its application, giving developers the freedom and flexibility to build high-performing and customizable user interfaces.
Virtual DOM
The Virtual DOM is an offshoot of the original or actual DOM that a web browser typically uses. While the browser’s DOM can be sluggish and inefficient when handling even minor state changes, React has devised a unique solution. It involves creating a second instance of the actual DOM with JavaScript, which is much faster and more responsive.
Each time a state change occurs, React utilizes this new “virtual” DOM to compare with the previous version, checking for differences. If the comparison shows that the two are identical or nearly identical, then the actual, browser-based DOM is never touched. Instead, only the necessary virtual DOM updates will be used to modify the actual DOM, resulting in a faster and more efficient process. This feature enables React to deliver an unparalleled level of performance and speed for web applications, regardless of their complexity.
Advantages of React
Increased efficiency and performance
React uses a Virtual DOM (VDOM) which improves rendering performance by reducing the number of direct manipulations in the original DOM. This allows users to quickly view dynamic data without the lag that can slow down traditional web page development.
Reusable Code Components
React uses a modular approach, meaning code written in one part of an application can be utilized in other parts of the application. This makes coding more efficient by allowing developers to reuse well-designed and thoroughly tested components.
Large Community and Strong Ecosystem
React has been around for over five years and has since gained a massive following. Its community is supported by several React-oriented libraries, frameworks, and tools, making it easier for developers and designers to explore the technology and learn as they go.
Easy Learning Curve
React has a simple and intuitive syntax, making it easier for beginners to grasp and more comfortable for experienced developers to use. Furthermore, its capacity to reuse code components enhances the process, making it easier for beginners to build their applications.
Disadvantages of React
Steep Initial Learning Curve
Though React has a low learning curve to get started, it may take time for beginners to grasp the nuances of handling states and props, a crucial part of React’s component model. Additionally, the application of JSX can also be problematic for developers uncomfortable with HTML elements.
Frequent and Abrupt Update Cycles
React has a rapid release cycle, and developers must keep up with the updates to stay up to date. A lack of familiarity with the latest updates and features might create difficulty for developers without any update training or experience.
No Standard, Prescriptive Template
React doesn’t provide standard templates or a prescriptive, ready-made directory for the application, leading to developers creating their own architectures and templates.
Requires Knowledge of Additional Tools
To work with React effectively, developers need to understand a multitude of additional tools, including build systems, bundlers, and compilers.
What Is Next.js
Next.js is a nimble framework that has been developed atop React, making it a breeze to craft swift, server-rendered websites. The framework came into being through the team at Vercel, and has been open-sourced right from the start.
It’s worth noting that Next.js is an immensely popular choice among some of the biggest players in the tech industry, including Airbnb, Twitter, and Uber. One of the standout advantages of Next.js is its inherent ability to automatically partition your application’s code, ensuring that each page only loads the requisite JavaScript needed for that particular view. This, in turn, leads to expeditious page loading times and a superior user experience.
Next.js also offers effortless compatibility with React Hooks, permitting you to employ stateful components with no supplementary setup.
Essential Features of Next.js
These features give React a leg up over competing frameworks:
- Type script support
- API route
- File system routing
- Static site generator
- Image optimization
- Automatic code splitting
- Server Side rendering
Type script support
TypeScript is a powerful superset of JavaScript that adds static typing, classes, and interfaces to the language. With Next.js, developers can take advantage of TypeScript’s many benefits, including improved code maintainability, easier debugging, and more reliable code.
API route
Next.js comes with a built-in API route that allows developers to create serverless functions that can be called directly from the client-side code. This feature makes it easy to build scalable and flexible web applications that can handle complex data operations.
File system routing
Next.js simplifies routing by allowing developers to define routes using the file system. This feature makes it easier to organize the code and makes the application more modular and scalable.
Static site generator
Next.js also supports static site generation, which means that the server can generate HTML pages at build time rather than waiting for a user to request a page. This feature can significantly improve website performance and user experience.
Image optimization
Next.js comes with built-in image optimization, which automatically compresses and resizes images to improve website performance. This feature makes it easy to use high-quality images without sacrificing website speed.
Automatic code splitting
Next.js automatically code-splits your application, meaning that each page only loads the necessary JavaScript for that page view. This results in faster page loads and an improved user experience.
Server Side rendering
One of the key advantages of Next.js is its support for server-side rendering. This means that the server can render the initial HTML of the page before sending it to the client’s browser, resulting in faster page loads and better search engine optimization.
Advantages of Next.js
Faster Page Loading
One of the key advantages of Next.js is its ability to automatically partition your application’s code, meaning that each page only loads the necessary JavaScript required for that particular view. This leads to faster page loads and a better user experience.
Server-Side Rendering
Next.js offers server-side rendering out of the box, which means that your website can be rendered on the server before being sent to the client’s browser. This ensures that your website is accessible to all users, regardless of their device or internet connection.
Simplified Routing
Next.js has a simple routing system that allows you to define dynamic routes without any additional configuration. This makes it easier to develop and maintain complex web applications.
React Integration
Next.js has seamless integration with React, which means that developers can use all the features of React, including React Hooks, without any additional setup.
Disadvantages of Next.js
Steep Learning Curve
Although Next.js is built on top of React, it has a learning curve of its own. Developers need to understand server-side rendering and the additional features offered by Next.js, which can take some time.
Limited Flexibility
Next.js is a framework that comes with its own set of rules and limitations. While it simplifies the development process, it can also limit the flexibility of developers who want to create highly customized web applications.
Limited Community
Although Next.js is gaining popularity, its community is still relatively small when compared to other web development frameworks. This can make it harder to find resources and get support when needed.
React compared to Next.js
In the world of front-end development, choosing the right tool is crucial. We’ll compare two of the most popular tools in the JavaScript ecosystem: Next.js and React, to help you make an informed decision about which tool to use based on your requirements.
Benchmark | React | Next.js |
---|---|---|
Documentation | As a widely-used library developed by Facebook, React has extensive and well-maintained documentation. The official documentation covers various aspects of React, including its core concepts, components, hooks, and best practices. Additionally, there is a vast number of tutorials, blog posts, and videos created by the developer community. | Next.js is built on top of React and provides additional features for server-side rendering and static site generation. Its official documentation is well-written, clear, and concise, making it easy to follow for both beginners and experienced developers. However, the amount of community-generated content is comparatively smaller than React. |
Configuration | React itself is a lightweight library and doesn’t impose any specific structure or configuration. However, setting up a production-ready React application may require additional configuration for bundling, transpiling, and routing. Tools like Create React App or custom webpack configurations can help streamline the process, but can sometimes be overwhelming for newcomers. | Next.js is designed to be more opinionated, offering a zero-configuration setup for most use cases. It automatically handles bundling, transpiling, and routing, allowing developers to focus on writing code. Customization is also possible, but typically requires less effort than in a React-only setup. |
State of Education | Given its popularity, there is no shortage of educational resources for React, including online courses, workshops, and tutorials. Many institutions and coding bootcamps include React in their curriculum, making it easier for developers to learn the library. | While educational resources for Next.js are growing, they are not as abundant as those for React. However, since Next.js is built on React, learning React first can provide a solid foundation before diving into Next.js-specific concepts. |
Performance | React is known for its efficient virtual DOM and component-based architecture, which promotes faster rendering and improved performance in most use cases. However, performance optimization for large-scale applications may require manual intervention and fine-tuning. | Next.js enhances React’s performance capabilities with automatic code splitting, server-side rendering (SSR), and static site generation (SSG). These features can lead to improved load times and SEO benefits for your application. |
Server-Side Rendering (SSR) | React is primarily a client-side rendering library, and implementing server-side rendering can be complex and time-consuming. It may require additional configurations, tools, and libraries like ReactDOMServer. | Next.js was built with server-side rendering in mind and makes it easy to implement SSR in your application. By default, Next.js pages are server-rendered, which can help improve performance, especially for SEO-critical applications. |
Is Next.js Better than React?
The answer to this question depends on your specific requirements. React is a client-side library used to build user interfaces, while Next.js is a framework built on top of React that provides additional features for server-side rendering and routing.
React is a lightweight library that offers great flexibility and control over the UI. It is widely used by developers and has a large community, which means that there are many resources and libraries available to extend its functionality. React is also known for its performance and efficient rendering of components.
Next.js, on the other hand, offers server-side rendering out of the box, which means that the application’s HTML pages are generated on the server before being sent to the client. This can significantly improve the performance of the application and is particularly useful for SEO. Next.js also includes features such as automatic code splitting and static site generation, which can further improve the performance and speed of the application.
When to Use React Over Next.js?
If you are building a client-side web application and don’t require server-side rendering, then React is a great choice. React is simple to use and can be easily integrated with other libraries and frameworks. React’s lightweight nature also means that it is ideal for building small to medium-sized applications.
React is particularly useful when you require a high level of interactivity on your website, such as user input and dynamic content. React’s virtual DOM allows it to update the UI efficiently, resulting in a smoother user experience.
When to Use Next.js Over React?
f you require server-side rendering or better SEO for your application, then Next.js is a better choice. Next.js offers server-side rendering out of the box, which means that the application’s HTML pages are generated on the server before being sent to the client. This can significantly improve the performance and SEO of your application.
Next.js also includes features such as automatic code splitting and static site generation, which can further improve the performance and speed of the application. Next.js is particularly useful for building large, content-heavy websites such as blogs or e-commerce websites.
Will Next.js Replace React
No, Next.js will not replace React. Next.js is built on top of React and offers additional features for server-side rendering and routing. While Next.js provides additional functionality, React remains the core library for building UI components in both client-side and server-side applications.
Conclusion
The choice between React and Next.js depends on the specific requirements of your project. React is a great choice for building client-side applications with a high level of interactivity. Next.js is a better choice for applications that require server-side rendering and improved SEO. Ultimately, both React and Next.js are powerful tools that can help you build high-quality web applications quickly and efficiently.